- Tkinter Tutorial Python
- Inverse Tkinter Tutorial Python
- Programming Python Pdf Download
- Tkinter Tutorial Python Pdf Download Pdf
- Download Tkinter For Python 2.7
- Tkinter Tutorial Python 3
• Tkinter is not necessarily turned on by default on your system. You can determine whether Tkinter is available for your Python interpreter by attempting to import the Tkinter module (in Python 1 and 2; renamed to tkinter in Python 3). If Tkinter is available, then no errors occur, as demonstrated in the following: >>> import tkinter >>.
Where can I find the most modern tutorial that teaches tkinter
together with ttk
?
Tkinter
seems the only way to go in Python 3 (don't suggest Python 2), and ttk
gave me hope for good-looking GUI.
closed as off-topic by Cristian Ciupitu, Bill the LizardJun 2 '14 at 15:34
This question appears to be off-topic. The users who voted to close gave this specific reason:
- 'Questions asking us to recommend or find a tool, library or favorite off-site resource are off-topic for Stack Overflow as they tend to attract opinionated answers and spam. Instead, describe the problem and what has been done so far to solve it.' – Bill the Lizard
4 Answers
I have found the TkDocs tutorial to be very useful. It describes building Tk
interfaces using Python and Tkinter
and ttk
and makes notes about differences between Python 2 and 3. It also has examples in Perl, Ruby and Tcl, since the goal is to teach Tk itself, not the bindings for a particular language.
Tkinter Tutorial Python
I haven't gone through the whole thing from start to finish, rather have only used a number of topics as examples for things I was stuck on, but it is very instructional and comfortably written. Today reading the intro and first few sections makes me think I will start working through the rest of it.
Finally, it's current and the site has a very nice look. He also has a bunch of other pages which are worth checking out (Widgets, Resources, Blog). This guy's doing a lot to not only teach Tk, but also to improve people's understanding that it's not the ugly beast that it once was.
nbroI recommend the NMT Tkinter 8.5 reference.
The module names used in some examples are those used in Python 2.7.
Here's a reference for the name changes in Python 3: link
One of the conveniences of ttk is that you can choose a preexistingtheme,
which is a full set of Styles applied to the ttk widgets.
Here's an example I wrote (for Python 3) that allows you to select any available theme from a Combobox:
Side note: I've noticed that there is a 'vista' theme available when using Python 3.3 (but not 2.7).
Inverse Tkinter Tutorial Python
Honest AbeHonest AbeI recommend reading the documentation. It is simple and authoritative, and good for beginners.
vy32vy32It's not really fresh but this is concise, and from what I've seen valid either for Python 2 and 3.
nbroNot the answer you're looking for? Browse other questions tagged python-3.xtkinterttk or ask your own question.
In this tutorial, we will learn how to develop graphical user interfaces by writing some Python GUI examples using the Tkinter package.
Join the DZone community and get the full member experience.
Join For FreeIn this tutorial, we will learn how to develop graphical user interfaces by writing some Python GUI examples using the Tkinter package.
Tkinter package is shipped with Python as a standard package, so we don't need to install anything to use it.
Tkinter is a very powerful package. If you already have installed Python, you may use IDLE which is the integrated IDE that is shipped with Python, this IDE is written using Tkinter. Sounds Cool!!
We will use Python 3.6, so if you are using Python 2.x, it's strongly recommended to switch to Python 3.x unless you know the language changes so you can adjust the code to run without errors.
I assume that you have a little background in the Python basics to help you understand what we are doing.
We will start by creating a window to which we will learn how to add widgets such as buttons, combo boxes, etc. Then we will play with their properties, so let's get started.
Create Your First GUI Application
First, we will import THE Tkinter package and create a window and set its title:
The result will look like this:
Awesome! Our application works.
The last line calls the mainloop
function. This function calls the endless loop of the window, so the window will wait for any user interaction till we close it.
If you forget to call the mainloop
function, nothing will appear to the user.
Create a Label Widget
To add a label to our previous example, we will create a label using the label class like this:
Then we will set its position on the form using the grid
function and give it the location like this:
So the complete code will be like this:
And this is the result:
Without calling the grid
function for the label, it won't show up.
Set Label Font Size
You can set the label font so you can make it bigger and maybe bold. You can also change the font style.
To do so, you can pass the font
parameter like this:
Note that the font
parameter can be passed to any widget to change its font, thus it applies to more than just labels.
Great, but the window is so small, what about setting the window size?
Setting Window Size
We can set the default window size using the geometry
function like this:
The above line sets the window width to 350 pixels and the height to 200 pixels.
Let's try adding more GUI widgets like buttons and see how to handle button click events.
Adding a Button Widget
Let's start by adding the button to the window. The button is created and added to the window in the same way as the label:
So our window will be like this:
The result looks like this:
Note that we place the button on the second column of the window, which is 1. If you forget and place the button on the same column which is 0, it will show the button only, since the button will be on the top of the label.
Change Button Foreground and Background Colors
You can change the foreground of a button or any other widget using the fg property.
Also, you can change the background color of any widget using the bg property.
Now, if you tried to click on the button, nothing happens because the click event of the button isn't written yet.
Handle Button Click Event
First, we will write the function that we need to execute when the button is clicked:
Then we will wire it with the button by specifying the function like this:
btn = Button(window, text= 'Click Me', command=clicked)
Note that, we typed clicked
only not clicked()
with parentheses.
Now the full code will be like this:
And when we click the button, the result, as expected, looks like this:
Cool!
Get Input Using Entry Class (Tkinter Textbox)
In the previous Python GUI examples, we saw how to add simple widgets, now let's try getting the user input using the Tkinter Entry
class (Tkinter textbox).
You can create a textbox using Tkinter Entry
class like this:
Then you can add it to the window using a grid function as usual
So our window will be like this:
And the result will be like this:
Now, if you click the button, it will show the same old message, but what about showing the entered text on the Entry widget?
First, you can get entry text using the get
function. So we can write this code to our clicked function like this:
If you click the button and there is text in the entry widget, it will show 'Welcome to' concatenated with the entered text.
And this is the complete code:
Run the above code and check the result:
Awesome!
Every time we run the code, we need to click on the entry widget to set focus to write the text, but what about setting the focus automatically?
Set the Focus of the Entry Widget
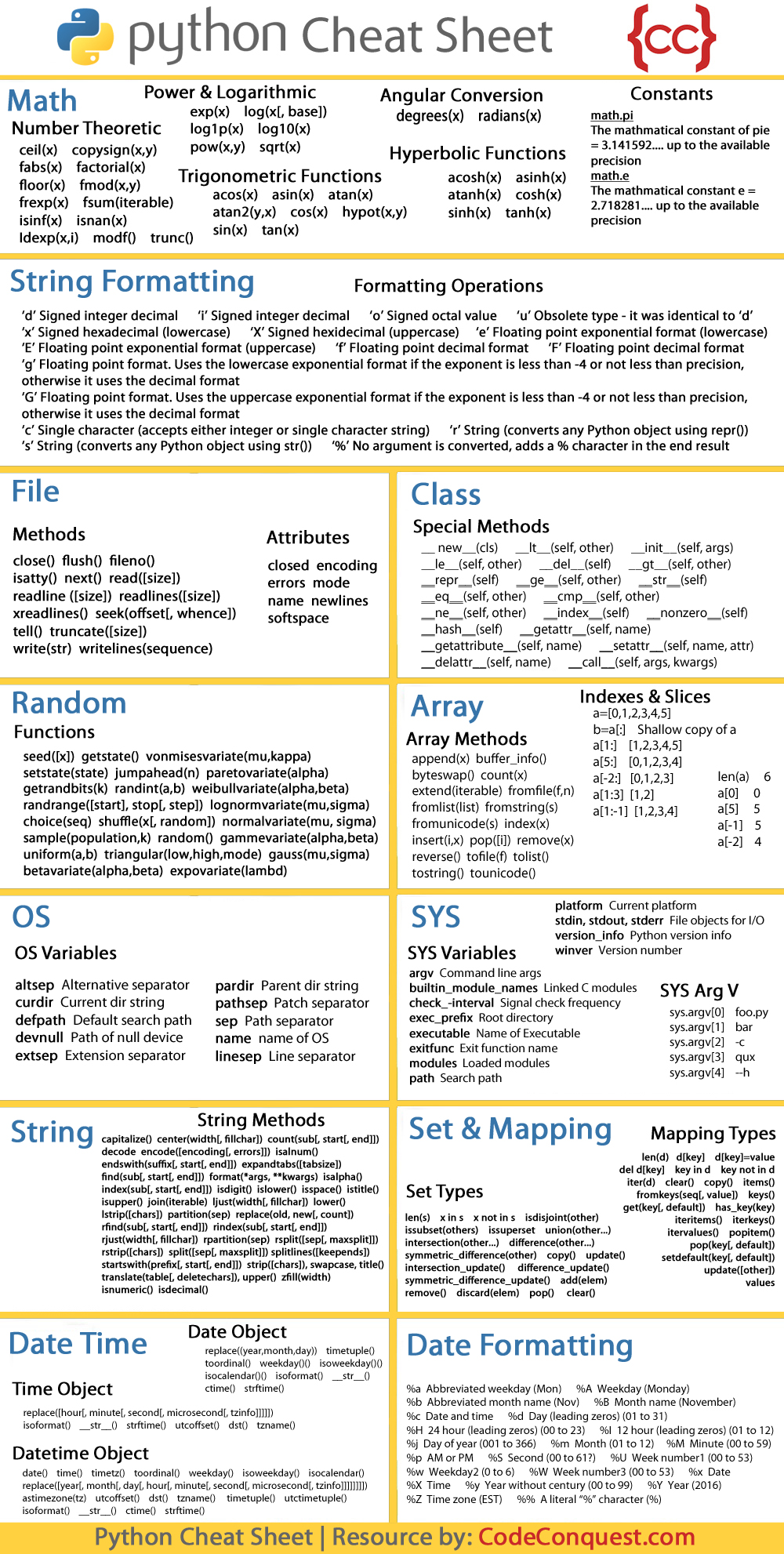
That's super easy, all we need to do is to call the focus
function like this:
And when you run your code, you will notice that the entry widget has the focus so you can write your text right away.
Disable the Entry Widget
To disable the entry widget, you can set the state property to disabled:
Now, you won't be able to enter any text.
Add a Combobox Widget
To add a combobox widget, you can use the Combobox
class from ttk library like this:
Then you can add your values to the combobox.
As you can see, we add the combobox items using the values tuple.
To set the selected item, you can pass the index of the desired item to the current function.
To get the select item, you can use the get
function like this:
Add a Checkbutton Widget (Tkinter Checkbox)
To create a checkbutton widget, you can use the Checkbutton
class like this:
Also, you can set the checked state by passing the check value to the Checkbutton like this:
Check the result:
Set the Check State of a Checkbutton
Here we create a variable of type BooleanVar
which is not a standard Python variable, it's a Tkinter variable, and then we pass it to the Checkbutton class to set the check state as the highlighted line in the above example.
You can set the Boolean value to false to make it unchecked.
Also, you can use IntVar
instead of BooleanVar
and set the value to 0 or 1.
These examples give the same result as the BooleanVar
.
Add Radio Button Widgets
To add radio buttons, you can use the RadioButton
class like this:
Note that you should set the value for every radio button with a different value, otherwise, they won't work.
The result of the above code looks like this:
Also, you can set the command of any of these radio buttons to a specific function, so if the user clicks on any one of them, it runs the function code.
This is an example:
Pretty simple!
Get Radio Button Values (Selected Radio Button)
To get the currently selected radio button or the radio button's value, you can pass the variable parameter to the radio buttons and later you can get its value.
Every time you select a radio button, the value of the variable will be changed to the value of the selected radio button.
Add a ScrolledText Widget (Tkinter textarea)
To add a ScrolledText widget, you can use the ScrolledText
class like this:
Here we specify the width and the height of the ScrolledText widget, otherwise, it will fill the entire window.
The result as you can see:
Set Scrolledtext Content
To set scrolledtext content, you can use the insert
method like this:
Delete/Clear Scrolledtext Content
To clear the contents of a scrolledtext widget, you can use the delete
method like this:
Great!
Create a Message Box
To show a message box using Tkinter, you can use the messagebox library like this:
Pretty easy!
Let's show a message box when the user clicks a button.
When you click the button, an informative message box will appear.
Show Warning and Error Messages
You can show a warning message or error message the same way. The only thing that needs to be changed is the message function
Show Ask Question Dialogs
To show a yes/no message box to the user, you can use one of the following messagebox
functions:
You can choose the appropriate message style according to your needs. Just replace the showinfo
function line from the previous line and run it.
Also, you can check what button was clicked using the result variable.
If you click OK or yes or retry, it will return True as the value, but if you choose no or cancel, it will return False.
The only function that returns one of three values is the askyesnocancel
function; it returns True or False or None.
Add a SpinBox (Numbers Widget)
To create a Spinbox widget, you can use the Spinbox
class like this:
Here we create a Spinbox widget and we pass the from_ and to parameters to specify the numbers range for the Spinbox.
Also, you can specify the width of the widget using the width parameter:
Check the complete example:
You can specify the numbers for the Spinbox instead of using the whole range like this:
Here the Spinbox widget only shows these 3 numbers: 3, 8, and 11.
Set a Default Value for Spinbox
To set the Spinbox default value, you can pass the value to the textvariable
parameter like this:
Now, if you run the program, it will show 36 as a default value for the Spinbox.
Add a Progressbar Widget
To create a progress bar, you can use the progressbar
class like this:
You can set the progress bar value like this:
You can set this value based on any process you want like downloading a file or completing a task.
Change Progressbar Color
Changing the Progressbar color is a bit tricky.
First, we will create a style and set the background color and finally set the created style to the Progressbar.
Check the following example:
And the result will look like this:
Add a File Dialog (File and Directory Chooser)
To create a file dialog (file chooser), you can use the filedialog
class like this:
After you choose a file and click open, the file variable will hold that file path.
Also, you can ask for multiple files like this:
Specify File Types (Filter File Extensions)
You can specify the file types for a file dialog using the filetypes
parameter, just specify the extensions in tuples.
You can ask for a directory using the askdirectory
method:
You can specify the initial directory for the file dialog by specifying the initialdir
like this:
Easy!
Add a Menu Bar
Programming Python Pdf Download

To add a menu bar, you can use the menu
class like this:
First, we create a menu, then we add our first label, and, finally, we assign the menu to our window.
You can add menu items under any menu by using the add_cascade()
function like this:
So our code will be like this:
This way, you can add as many menu items as you want.
Tkinter Tutorial Python Pdf Download Pdf
Here we add another menu item called Edit with a menu separator.
You may notice a dashed line at the beginning, well, if you click that line, it will show the menu items in a small separate window.
You can disable this feature by disabling the tearoff
feature like this:
Just replace the new_item
in the above example with this one and it won't show the dashed line anymore.
I don't need to remind you that you can type any code that works when the user clicks on any menu item by specifying the command property.
Add a Notebook Widget (Tab Control)
To create a tab control, there are a few steps.
- First, we create a tab control using the
Notebook
class. - Create a tab using the
Frame
class. - Add that tab to the tab control.
- Pack the tab control so it becomes visible in the window.
In this way, you can add as many tabs as you want.
Add Widgets to Notebooks
After creating tabs, you can put widgets inside these tabs by assigning the parent property to the desired tab.
Add Spacing for Widgets (Padding)
You can add padding for your controls to make it look well organized using the padx
and pady
properties.
Just pass padx
and pady
to any widget and give them a value.
It's that simple!
In this tutorial, we saw many Python GUI examples using the Tkinter library and we saw how easy it's to develop graphical interfaces using it.
This tutorial covered the main aspects of Python GUI development, but not all of them. There is no tutorial or a book that can cover everything.
I hope you found these examples useful. Keep coming back.
Thank you.
Like This Article? Read More From DZone
Download Tkinter For Python 2.7
Published at DZone with permission of Mokhtar Ebrahim , DZone MVB. See the original article here.
Tkinter Tutorial Python 3
Opinions expressed by DZone contributors are their own.